An array is a sequence of memory locations for storing data set out in such a way that any one of the individual locations can be accessed by quoting its index number.
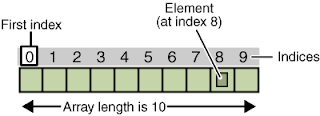
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the above illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
Declaring Java Arrays
To declare an array, write the data type, followed by a set of square brackets [ ], followed by the identifier name.
ElementType [ ] arrayName;
Example:
int [ ] age;
float grades [ ];
String name [ ];
Initializing Array Variables
To initialize an array, you use the new operator to allocate memory for objects. The new operator is followed by the data type with the number of elements to allocate specified the number of elements to be allocated is placed within the [ ] operator.
Example:
age = new int [5];
grades = new float [10];
name = new String [100];
Like other variables, an array variable can be initialized when it is declared.
ElementType[ ] arrayName = new ElementType [ sizeOfArray];
Example:
int [ ] age = new int [ 5 ];
float [ ] grades = new float [10];
String name [ ] = new String [100];
Sometimes user declares an array and it's size simultaneously. You may or may not be define the size in the declaration time. An array can be also created by directly initializing it with data.
Example:
int [ ] arr = {1, 2, 3, 4, 5};
This statement declares and creates an array of integers with five elements, and initializes this with the values 1, 2, 3, 4, and 5.
String [ ] days = {"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"};
This statement declares and creates an array of string with identifier days and initialized. This array contains 7 elements.
Accessing an Array Element
To access an array element, or a part of the array, use a number called an index or a subscript.
An index number or subscript assigned to each member of the array, to allow the program to access an individual member of the array.
It is an integer beginning at zero and progresses sequentially by whole numbers to the end of the array. Index is from 0 to (sizeOfArray - 1).
Example:
//assigns 5 to the first element in the array
age [0] = 5;
// prints the last element in the array
System.out.println(age[4]);
Here is the code of the program:
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a;
int num [] = new int[10];
for ( a=0; a<10;>> ");
num[a] = Integer.parseInt(x.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<10; style="font-weight: bold;">Sample Output:
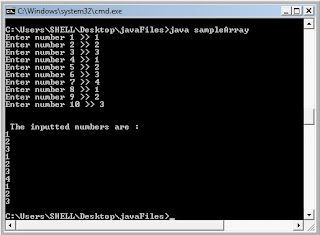
Try to create a program that will display the sum of 5 numbers using one dimensional array.
117 comments:
//Try to create a program that will display the sum of 5 numbers in one dimensional of array//
/*Jemar Christopher O. Cristobal BSCS-112*/
Good evening mam\/",)
e2 po comment ko
============***********============
import java.io.*;
public class sampleArrayjcoc
{
public static void main (String args []) throws IOException
{
BufferedReader jc=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0;a<5;a++)>> ");
sum[a] = Integer.parseInt(jc.readLine());
}
System.out.print("lnln The sum is : +sum ln")
}
}
//Tama po sana^^//
// kristine quiling,,DIT111 //
comment ko...?
basta maganda si mam rashell
import java.io.*;
Public class samplearray
{
Public Static Void Main (String args[])throws IOexception
{
BufferedReader in=new BufferedReader(new InputStreamReader(System.ini));
int a;
a=2
int num [] = new int[5];
do
{
num[a] = Integer.parseInt(in.readLine());
System.out.print(""+ num[a]+);
a=(a++);
}
while (a<6);
}
}
//eto po sa akin.. paki PM nmn po if me mali ^^//
//Thank you //
(wak nmn sana mali lahat na nmn Y_Y)
Nice mam!...ganda talaga ni mam! Ehehehehe!
public class comment1mfc
{
public static void main(String args[])
{
String name="midKNIGHTe";
System.out.print("ang galing talaga ni mam");
System.out.print("\n at ang ganda pa");
System.out.print("\n String :"+name);
}
}
this is the way i live..this is the way i am!!!
-midKNIGHTe (camonias)
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader jml=new BufferedReader (new InputStreamReader (System.in));
int a;
int num [] = new int[6];
for ( a=0; a<6; a++)
{
System.out.print ("Enter number " + (a+1) + " >> ");
num[a] = Integer.parseInt(jml.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<6; a++)
{
System.out.println("" + num[a]);
}
public class comment2aoa
{
public static void main(String args[])
{
String name="Devil aLvin";
System.out.print("mam da best ka talaga pano mo nagawa un galing hehehehe");
System.out.print("\n mam ganda mo talaga");
System.out.print("\n String :"+name);
}
}
ma'm YOUR MY GUARDIAN ANGEL!!!
+acesor+ 15
MAam,,.
anu ba sasabihin??
//Joy Kristine F. Tabunan BSCS 112//
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader jkft=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print ("Enter sum " + (a+1) + " >> ");
sum[a] = Integer.parseInt(jkft.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<5; a++)
{
System.out.println("" + sum[a]);
}
}
}
public class comment1JAB
{
public static void main(String args[])
{
String name="GORIO";
System.out.print("ang husay ni mam");
System.out.print("\n at cute pa");
System.out.print("\n String :"+name);
}
}
waaahhhh.. .kamoteness na naman ang aabutin q dito.. .
pnu ba yan??sana madali un.. .
"aray" in java programming hehehehe
Emerson Dejoras DIT-111
""""ang hrap nmn n2 pro ok lng bsta anjan c mam "ARCE ok lng hahahaha"""
nagaraya_15@hotmail.com
ma"am cnxa n poh d ko po kc masyadong maintindihan yong program...,
antayin q n lang po kayong maglecture...,
Urica LaspiƱas DIT111
import java.io.*;
public class sampleArraygfm
{
public static void main(String args[]) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader(System.in));
int g;
int a [] = new int[5];
for ( g=0; g<5; g++)
{
System.out.print ("Enter number ");
a[g] = Integer.parseInt(x.readLine());
}
System.out.print("\n\n The sum are \n");
for(g=0; g<10; g++)
{
System.out.println("" + a[g]);
}
}
}
gabriel magno DIT 113
mam di ko po masyado maintindihan yung program
michelle calapit
mam di ko mxado maintindihan eh.....
Rhodora Tagaya...DIT111
mam hindi ko maintindihan...................ira jastiva
hallow mam more explanation pa poh...............rochelle ocan
ma'am pahirap n ng phirap ang java.....d ko n masyado maintindihan.....hehe....
pero ok lang maganda naman prof. namen hehe.......
cecil dalawampo DIT-111
mam! mejo tama yung ginawa ko pero mam paliwanag niyo din po para tama na yung gaw ko po tnx mam!
mam .. . parang ang hirap naman . . .
hehehe. . p
pero ok lang . . maganda naman ako . . .
hehehe. .
myron habig . .
maam. baka nman pede discussion muna ang hirap poh talaga pag walang discussion nio cnxa na poh mostly walang nkagwa smen!!!!
jerico arenas>>
Navarro R. Rofiles
DIT 113
maayuz pa sa maayuz....
lufet....
import java.io.*;
public class ARRAYreu
{
public static void main (String args[]) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader(System.in));
int a;
int[]arr={1,2,3,4,5};
for (a=0;a<5;a++)
{
System.out.print ("Enter number: ");
a= Integer.parseInt(x.readLine());
}
for(a=0;a<5;a++)
{
System.out.print("\n The sum of the inputted numbers is "+a);
}
}
}
...umali 113...
Navarro R. Rofiles
DIT 113
import java.io.*;
public class ArrayNRR
{
public static void main (String args []) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a;
int num [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print ("Enter number " + (a+1) + " : ");
num[a] = Integer.parseInt(x.readLine());
}
System.out.print("\n The inputted numbers are : \n");
for(a=0; a<5; a++)
{
System.out.println("" + num[a]);
}
}
}
import java.io.*;
public class sampleArray1ets
{
public static void main (String args []) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a;
int num[]=new int[5];
for ( a=0; a<5; a++)
{
System.out.print ("Enter number " + (a+1) + " >> ");
num[a] = Integer.parseInt(x.readLine());
}
System.out.print("\n\n The sum of the inputted numbers are : \n");
for(a=0; a<5; a++)
{
System.out.println("" + num[a]);
}
}
}
erika bianca sarmiento DIT113
ma'am ang hirap sakit sa ulo.pero my natutunan naman ako eheheheh
Hi ma'm paturo na lang po sa lecture^^,..
JLor
Mark Anthony Flores
DIT113
\\* Thanks Ma'am n_n
eto po sagot ko sumakit ulo ko jan
sana tama kahit alam kong mali:
sobra ng isang count huhuhu :'(
import java.io.*;
public class array1mrf
{
public static void main (String args []) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a,mrf;
mrf=0;
a=0;
int num [] = new int[5];
do
{System.out.print ("Enter number " + (mrf+1) + " = ");
num[mrf] = Integer.parseInt(x.readLine());
num[a] = num[mrf] + num[a];
mrf++;}
while (mrf<5);
System.out.print("The Sum is Equals to: " +num[a]);
}
}
mam dq po maxado maintndihan ang ARRAY....
Angelo Pelaez
Try ko sana gawin kaya lng wala ng oras lapit na magklase tau ma'm^^
import java.io.*;
public class arraycsm
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int csm;
int sum[a] = new int[5];
do
{
System.out.print("Enter a number "+(csm=+1) + "=");
}
{sum=[csm]=Integer.parseInt(x.readLine());
num=[a]=num[a]+num[csm];
csm++;}
while (csm<=5)
System.out.print("The sum is equals to: "+sum[a]);
}
}
caren mendoza DIT 113ma'am d koh lang poh alam kng tma..tnx ma'am
lyann gavarra BSCS113
_try to create a program that will display the sum of 5 numbers in one dimensional of array_
_haAy!!sana po tama gawa ko_
. . . . . . . . . . . . . . . . . .
import java.io.*;
public class Samplearraylpg
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int a;
int sum[]=new int[5];
for(a=5;a<5;a++)
{
System.out.print("\nenter number" +(a+5)+">>");
sum[a]=Integer.parseInt(x.readLine());
}
{
System.out.print("\nthe sum is" + sum);
}
}
}
mam sorry po d ko po maintndhan eh,,,mki2ng n lng po ako 2m ng discuss m,,tke care po...(-,-).....
Lovely A. Leynes
DIT 111
mam di kopo maintndhan,,,,,
sorry po.....thak u. makikinig nalang po ako pag nag discuss po kayo.
crestita s. ramo
import java.io.*;
public class array1janna
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum []=new int[5];
for (a=5;a<5;a++);
{
System.out.print("Enter Number"+(a+5)+">>");
sum[a]=Integer.parseInt(x.readLine());
}
{
System.out.print("The sum of numbers is "+sum);
}
}
}
wohh!wah kame ma say!..maAm paChEzz Burger NmaN jAN!!ahehe!
=]good pm poh...
eto po yun sgot koh..
Try lang poh..
i hope tama...
-----------------------------------
Try to create a program that will display the sum of 5 numbers using one dimensional array.
-----------------------------------
public class sampleApril
{
public static void main (String args []) throws IOException
{
BufferedReader April=new BufferedReader (new InputStreamReader (System.in));
int a;
int [ ] num = {1, 2, 3, 4, 5};
while (a<5)
sum[a] = Integer.parseInt(april.readLine());
}
System.out.print("This is the sum: +sum ")
}
}
Created by:APRILYN D. BAET BSCS111
Ms. RASHELL ARCE
-----------------------------------
KEEP UP THE GOOD WORK MAM'
KHIT MAHIRAP JAVA..
i'll TRy my best poh..
GODBLESS..
=]good pm poh...
eto po yun sgot koh..
Try lang poh..
i hope tama...
-----------------------------------
Try to create a program that will display the sum of 5 numbers using one dimensional array.
-----------------------------------
public class sampleApril
{
public static void main (String args []) throws IOException
{
BufferedReader April=new BufferedReader (new InputStreamReader (System.in));
int a;
int [ ] num = {1, 2, 3, 4, 5};
while (a<5)
sum[a] = Integer.parseInt(april.readLine());
}
System.out.print("This is the sum: +sum ")
}
}
Created by:APRILYN D. BAET BSCS111
Ms. RASHELL ARCE
-----------------------------------
KEEP UP THE GOOD WORK MAM'
KHIT MAHIRAP JAVA..
i'll TRy my best poh..
GODBLESS..
=]good pm poh...
eto po yun sgot koh..
Try lang poh..
i hope tama...
-----------------------------------
Try to create a program that will display the sum of 5 numbers using one dimensional array.
-----------------------------------
public class sampleApril
{
public static void main (String args []) throws IOException
{
BufferedReader April=new BufferedReader (new InputStreamReader (System.in));
int a;
int [ ] num = {1, 2, 3, 4, 5};
while (a<5)
sum[a] = Integer.parseInt(april.readLine());
}
System.out.print("This is the sum: +sum ")
}
}
Created by:APRILYN D. BAET BSCS111
Ms. RASHELL ARCE
-----------------------------------
KEEP UP THE GOOD WORK MAM'
KHIT MAHIRAP JAVA..
i'll TRy my best poh..
GODBLESS..
=]good pm poh...
eto po yun sgot koh..
Try lang poh..
i hope tama...
-----------------------------------
Try to create a program that will display the sum of 5 numbers using one dimensional array.
-----------------------------------
public class sampleApril
{
public static void main (String args []) throws IOException
{
BufferedReader April=new BufferedReader (new InputStreamReader (System.in));
int a;
int [ ] num = {1, 2, 3, 4, 5};
while (a<5)
sum[a] = Integer.parseInt(april.readLine());
}
System.out.print("This is the sum: +sum ")
}
}
Created by:APRILYN D. BAET BSCS111
Ms. RASHELL ARCE
-----------------------------------
KEEP UP THE GOOD WORK MAM'
KHIT MAHIRAP JAVA..
i'll TRy my best poh..
GODBLESS..
=]good pm poh...
eto po yun sgot koh..
Try lang poh..
i hope tama...
-----------------------------------
Try to create a program that will display the sum of 5 numbers using one dimensional array.
-----------------------------------
public class sampleApril
{
public static void main (String args []) throws IOException
{
BufferedReader April=new BufferedReader (new InputStreamReader (System.in));
int a;
int [ ] num = {1, 2, 3, 4, 5};
while (a<5)
sum[a] = Integer.parseInt(april.readLine());
}
System.out.print("This is the sum: +sum ")
}
}
Created by:APRILYN D. BAET BSCS111
Ms. RASHELL ARCE
-----------------------------------
KEEP UP THE GOOD WORK MAM'
KHIT MAHIRAP JAVA..
i'll TRy my best poh..
GODBLESS..
Try to create a program that will display the sum of 5 numbers using one dimensional array.
cristopher ryan b perez dit112 said...
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader jml=new BufferedReader (new InputStreamReader (System.in));
int a;
int num [] = new int[6];
for ( a=0; a<6; a++)
{
System.out.print ("Enter number " + (a+1) + " >> ");
num[a] = Integer.parseInt(jml.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<6; a++)
{
System.out.println("" + num[a]);
}
jumar moreno de torres
dit112
progfun
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader jml=new BufferedReader (new InputStreamReader (System.in));
int a;
int num [] = new int[6];
for ( a=0; a<6; a++)
{
System.out.print ("Enter number " + (a+1) + " >> ");
num[a] = Integer.parseInt(jml.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<6; a++)
{
System.out.println("" + num[a]);
}
mark anthony Morillo> DIT112
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader mark=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum []=new int[5];
for (a=0; a<5; a++)
{
System.out.print ("Enter sum " + (a+1) + " >> ");
sum[a] = Integer.parseInt(mark.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<5; a++)
{
System.out.println("" + sum[a]);
}
}
}
mam ito po ung sakin sana po tama
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader c=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print ("Enter sum " + (a+1) + " >> ");
sum[a] = Integer.parseInt(c.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<5; a++)
{
System.out.println("" + sum[a]);
}
}
}
Ronald Nanez BSIT 112
import java.io.*;
public class sampleArray
{
public static void main(String args[]) throws IOException
{
BufferedReader in=new BufferedReader (new InputStreamReader(System.in));
int g;
int a [] = new int[5];
for ( g=0; g<5; g++)
{
System.out.print ("Enter number ");
a[g] = Integer.parseInt(in.readLine());
}
System.out.print("\n\n The sum are \n");
for(g=0; g<10; g++)
{
System.out.println("" + a[g]);
}
Helbert Subaan BSCS 112
____Jervy P. Sardona___c
import java.io.*;
public class sampleArrayjps
{
public static void main (String args []) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0;a<5;a++)>> ");
sum[a] = Integer.parseInt(x.readLine());
}
System.out.print("lnln The sum is : +sum ln")
}
}
_____________mAm e2 po ung akin___
___tnx mam__ingat po___gud evea--_
mport java.io.*;
public class sampleArraygfm
{
public static void main(String args[]) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader(System.in));
int a,b;
for ( a=0; b<5; g++)
{
System.out.print ("Enter number ");
a[b] = Integer.parseInt(x.readLine());
}
System.out.print("\n\n The sum are \n");
for(b=0; b<10; g++)
{
System.out.println(" + a[b]");
}
}
}
<<<< Nicole John G. Viray
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader in=new BufferedReader (new InputStreamReader (System.in));
int a,b,c,d,e,f;
int num [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print("Enter number:");
num[b]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[c]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[d]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[e]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[f]=Integer.parseInt(in.readLine());
sum=b+c+d+e+f;
}
System.out.println("" + num[sum]);
}
}
}
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader in=new BufferedReader (new InputStreamReader (System.in));
int a,b,c,d,e,f;
int num [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print("Enter number:");
num[b]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[c]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[d]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[e]=Integer.parseInt(in.readLine());
System.out.print("Enter number:");
num[f]=Integer.parseInt(in.readLine());
sum=b+c+d+e+f;
}
System.out.println("" + num[sum]);
}
}
}
hehe maaM anG hiRAP nYan pinagiCpan Q TALAga YAn kahT maLi
LagYAn nio nMn pO gRadE kahT 75
he3!!
WaG niOnG paGtaWanan maam Ung pRog Q hUh?
JACOB MANCENIDO
BSIT-112
Sagot ko o!
import java.io.*;
public class array1mrf
{
public static void main (String args []) throws IOException
{
BufferedReader Jacob=new BufferedReader (new InputStreamReader (System.in));
int a,j;
j=0;
a=1;
int num [] = new int[5];
do
{System.out.print ("Enter number " + (j+1) + ": ");
num[j] =Integer.parseInt(Jacob.readLine());
num[a] = num[j] + num[a];
j++;
}
while (j<5);
System.out.print("The Sum is : " +num[a]);
}
}
--,.TAMA sanA.,--
Ma'am Add me up d8o_025@yahoo.com pls. pls. pls.! =)!
JACOB MANCENIDO
BSIT-112
Sagot ko o!
import java.io.*;
public class array1mrf
{
public static void main (String args []) throws IOException
{
BufferedReader Jacob=new BufferedReader (new InputStreamReader (System.in));
int a,j;
j=0;
a=1;
int num [] = new int[5];
do
{System.out.print ("Enter number " + (j+1) + ": ");
num[j] =Integer.parseInt(Jacob.readLine());
num[a] = num[j] + num[a];
j++;
}
while (j<5);
System.out.print("The Sum is : " +num[a]);
}
}
--,.TAMA sanA.,--
Ma'am Add me up d8o_025@yahoo.com pls. pls. pls.! =)!
JACOB MANCENIDO
BSIT-112
Sagot ko o!
import java.io.*;
public class array1mrf
{
public static void main (String args []) throws IOException
{
BufferedReader Jacob=new BufferedReader (new InputStreamReader (System.in));
int a,j;
j=0;
a=1;
int num [] = new int[5];
do
{System.out.print ("Enter number " + (j+1) + ": ");
num[j] =Integer.parseInt(Jacob.readLine());
num[a] = num[j] + num[a];
j++;
}
while (j<5);
System.out.print("The Sum is : " +num[a]);
}
}
--,.TAMA sanA.,--
Ma'am Add me up d8o_025@yahoo.com pls. pls. pls.! =)!
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader anzem=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print ("Enter number " + (a+1) + " >> ");
sum[a] = Integer.parseInt(anzem.readLine());
}
System.out.println("Sum = " +sum);
}
}
//Try to create a program that will display the sum of 5 numbers in one dimensional of array//
/*Jemar Christopher O. Cristobal BSCS-112*/
import java.io.*;
public class sampleArrayjcoc
{
public static void main (String args []) throws IOException
{
BufferedReader jc=new BufferedReader (new InputStreamReader (System.in));
int num[]= new int[5];sum=0;
for(int jc=0;jc<5;jc++)
{
System.out.print("Enter a Number: ");
num[jc]=Integer.parseInt(jc.readLine());
sum=sum+num[jc];
}
System.out.print("Sum: "+sum);
}
}
KONBANWA SENSEI-SAMA
(Goodevening Maam)
sana po tama n ung sinagutan ko....
\/",) syanga pala maam,,,,
d ka sumama nung saturday
sayang ng JAVA sana tau s pool..^^
cge po sna po tlga tama na..
ANATA NO BLOPSPOT-WA KAWAI NEH
(your blogspot is beautiful^^)
//Try to create a program that will display the sum of 5 numbers in one dimensional of array//
< Jerick I. Bayotas > (,")
Gud eve po Mam,, ^_^
i2 po comment q!!
<<<<<<<<<<<<__________>>>>>>>>>>>
import java.io.*;
public class sampleArrayJIB
{
public static void main(String args[])throws IOException
{
BufferedReader x = new BufferedReader (new InputStreamReader (System.in));
int a;
a=1;
int num [0] = new int[5];
while (a<6)
{
num[a] = Integer.parseInt(in.readLine());
}
a++;
System.out.print("lnln The sum is : +sum ln")
}
}
/*Franz Ian B. Alucilja BSIT 112*/
import java.io.*;
public class ARRAY
{
public static void main (String args []) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a, b;
int num [] = new int[10];
for ( a=0; a<5; a++)
{
System.out.print ("Enter number " + (a+1) + " >> ");
num[a] = Integer.parseInt(x.readLine());
}
b=0;
for(a=0; a<5; a++)
{
b=b + num[a];
a+1;
}
System.out.print("The sum of the numbers is: " + b);
}
}
//Try to create a program that will display the sum of 5 numbers in one dimensional of array//
< Reynald Bernie R. Medina_D.I.T.-112 >
import java.io.*;
public class sampleArrayRRM
{
public static void main(String args[])throws IOException
{
BufferedReader x = new BufferedReader (new InputStreamReader (System.in));
int a;
a=1;
int num [0] = new int[5];
while (a<6)
{
num[a] = Integer.parseInt(in.readLine());
}
a++;
System.out.print("lnln The sum is : +sum ln")
}
}
//Try to create a program that will display the sum of 5 numbers in one dimensional of array//
Reynald Bernie R. Medina-D.I.T.-112
import java.io.*;
public class sampleArrayRRM
{
public static void main(String args[])throws IOException
{
BufferedReader x = new BufferedReader (new InputStreamReader (System.in));
int a;
a=1;
int num [0] = new int[5];
while (a<6)
{
num[a] = Integer.parseInt(in.readLine());
}
a++;
System.out.print("lnln The sum is : +sum ln")
}
}
import java.io.*;
public class Array1
{
public static void main (String args []) throws IOException
{
BufferedReader aki=new BufferedReader (new InputStreamReader (System.in));
int sum;
int num [] = new int[5];
int a=0;
for(a=0;a<5;a++)
{
System.out.print("Enter number:");
num[a]=Integer.parseInt(aki.readLine());
sum+=num[a];
}
System.out.print("The sum is " + sum);
}
}
ma'am that was my analysis . . . sana po tama . . . nosebleed po ako jan ^_^. . . cnxa po late ko na naipost . . . nageerror ang net po sa bahay kagabi . . . ma'am favor nmn po pa add na rin po ako sa ym para whenever na my itatanong po ako madali ko masasabi . . . linkonkev@y.c.ph
yan po . . . thank you so much po^^. . .
import java.io.*;
public class sampleArrayjime
{
public static void main(String args[]) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader(System.in));
int b;
int a [] = new int[5];
for ( b=0; b<5; b++)
{
System.out.print ("Enter number ");
a[b] = Integer.parseInt(x.readLine());
}
System.out.print("\n\n The sum are \n");
for(b=0; b<10; b++)
{
System.out.println("" + a[b]);
}
}
}
import java.io.*;
public class sampleArrayjime
{
public static void main(String args[]) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader(System.in));
int b;
int a [] = new int[5];
for ( b=0; b<5; b++)
{
System.out.print ("Enter number ");
a[b] = Integer.parseInt(x.readLine());
}
System.out.print("\n\n The sum are \n");
for(b=0; b<10; b++)
{
System.out.println("" + a[b]);
}
}
}
Mariel Alpay DIT-111
hi mam.. .ganda ng ginawa mo array
import java.io.*;
public class ARRAYrcr
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int z;
int sum [] = new int [5]
for (z=0;z<=5;z++);
{
System.out.print("Enter a number");
z=Integer.parseInt(x.readLine());
{for (z=0;z<=5;z++);
System.out.print("\n The sum of the inputted numbers is "+z);
}
}
}
Ramos DIT112
System.out.print
import java.io.*;
public class sampleArrayjcoc
{
public static void main (String args []) throws IOException
{
BufferedReader jc=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0;a<5;a++)>> ");
sum[a] = Integer.parseInt(jc.readLine());
}
System.out.print("lnln The sum is : +sum ln")
}
}
}
import java.io.*;
public class sampleArrayjcoc
{
public static void main (String args []) throws IOException
{
BufferedReader jc=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0;a<5;a++)>> ");
sum[a] = Integer.parseInt(jc.readLine());
}
System.out.print("lnln The sum is : +sum ln")
}
}
}
import java.io.*
public class sample arrayJRP
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int a;
int sum [] = new int [5];
for (a=0;a<=5;a++);
{
System.out.print("Enter number " + (a+1) + " >> ");
{
System.out.print("("This is the sum: +sum ")
}
}
import java.io.*
public class sample arrayJRP
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int a;
int sum [] = new int [5];
for (a=0;a<=5;a++);
{
System.out.print("Enter number " + (a+1) + " >> ");
{
System.out.print("("This is the sum: +sum ")
}
}
Jenny-lyn Parcon DIT112
import java.io.*;
public class sampleArrayjcoc
{
public static void main (String args []) throws IOException
{
BufferedReader jc=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0;a<5;a++)>> ");
sum[a] = Integer.parseInt(jc.readLine());
}
System.out.print("lnln The sum is : +sum ln")
}
}
import java.io.*;
public class sampleArray
{
public static void main(String args) throw IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int a;
int sum []=new int[5];
for( a=0;a<5;a++)>> ");
sum[a] = Integer.parseInt(x.readLine());
}
System.out.print("The sum is" +sum);
}
}
}
[=JOralie Santayana DIT112=]
ma'am eto po sagot ko sa problem... sana po tama!!!,
PROBLEM:Create a program that will accept 10 floating numbers, compute and display the average. Class name is Ass1xxx.
Solution:
import java.io.*;
public class Ass1ual
{
public static void main (String args []) throws IOException
{
float u,r,i,l;
u=0; r=0; l=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
i=Float.parseFloat(x.readLine());
u=u+i;}
l=u/10;
System.out.print ("Average is: "+l);
}
}
Urica A. LaspiƱas
DIT111
import java.io.*;
public class ARRAYsel
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int a ;
int sum [] = new int [5]
for (a=0;a<=5;a++);
{
System.out.print("Enter a number");
a=Integer.parseInt(x.readLine());
{for (a=0;a<=5;a++);
System.out.print("\n The sum:+sum ln);
}
}
}
import java.io.*;
public class ARRAYsel
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int a ;
int sum [] = new int [5]
for (a=0;a<=5;a++);
{
System.out.print("Enter a number");
a=Integer.parseInt(x.readLine());
{for (a=0;a<=5;a++);
System.out.print("\n The sum:+sum ln);
}
}
}
_..sylvia lladoc dit 112.._
\\Create a program that will accept 10 floating numbers. Compute and display the average. Class name is ASS1xxx.
import java.io.*;
public class Ass1mfc
{
public static void main (String args []) throws IOException
{
float m,a,f,c;
m=0; a=0; c=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
f=Float.parseFloat(x.readLine());
m=m+f;
}
c=m/10;
System.out.print ("Average is: "+c);
}
}
midKNIGHTe (camonias)
DIT - 111
\\Create a program that will accept 10 floating numbers. compute and display the average. CLass name is ASS1xxx.
import java.io.*;
public class Ass1aoa
{
public static void main (String args []) throws IOException
{
float a,c,s,r;
a=0; c=0; r=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
s=Float.parseFloat(x.readLine());
a=a+s;}
r=a/10;
System.out.print ("Average is: "+r);
}
}
+Devil alvin+ (acesor)=15
DIT 111
import java.io.*;
public class sampleArraynso
{
public static void main(String args) throw IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int a;
int sum []=new int[6];
for( a=0;a>6;a++)<< ");
sum[a] = Integer.parseInt(x.readLine());
}
System.out.print("The sum is" +sum);
}
}
}
Ninio S.Obmerga
dit-112
ma'm absent poh ko last meeting kya d ko alam..tyuri.....copy paste poh ung sken.....khit naman poh andun ako d ko rin maiintndhan ang hrap pra sken ma'm....huhuhuhuhu.....tyuri poh>>>>>>
obmerga....
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader jkft=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print ("Enter sum " + (a+1) + " >> ");
sum[a] = Integer.parseInt(jkft.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<5; a++)
{
System.out.println("" + sum[a]);
}
}
Maricar Tuyo
Dit 112
import java.io.*;
public class sampleArray
{
public static void main (String args []) throws IOException
{
BufferedReader jkft=new BufferedReader (new InputStreamReader (System.in));
int a;
int sum [] = new int[5];
for ( a=0; a<5; a++)
{
System.out.print ("Enter sum " + (a+1) + " >> ");
sum[a] = Integer.parseInt(jkft.readLine());
}
System.out.print("\n\n The inputted numbers are : \n");
for(a=0; a<5; a++)
{
System.out.println("" + sum[a]);
}
}
Maricar Tuyo
Dit 112
\\Create a program that will accept 10 floating numbers. Compute and display the average. Class name is ASS1xxx.
import java.io.*;
public class Ass1djf
{
public static void main (String args []) throws IOException
{
float a,b,c,d;
a=0; b=0; d=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
c=Float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print ("Average is: "+d);
}
}
dan jewel fojas
dit 111
\\Create a program that will accept 10 floating numbers. Compute and display the average. Class name is ASS1xxx.
import java.io.*;
public class Ass1jca
{
public static void main (String args []) throws IOException
{
float a,b,c,d;
a=0; b=0; d=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
c=Float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print ("Average is: "+d);
}
}
Joseph Amparo
DIT 111
import java.io.*;
public class Ass1aoa
{
public static void main (String args []) throws IOException
{
float a,b,c,d;
a=0; b=0; d=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
c=Float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print ("Average is: "+d);
}
}
import java.io.*;
public class Ass1aoa
{
public static void main (String args []) throws IOException
{
float a,b,c,d;
a=0; b=0; d=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
c=Float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print ("Average is: "+d);
}
}
Angelo Pelaez
DIT 111
\\Create a program that will accept 10 floating numbers. Compute and display the average. Class name is ASS1xxx.
import java.io.*;
public class Ass1jab
{
public static void main (String args []) throws IOException
{
float a,b,c,d;
a=0; b=0; d=0;
for (r=0; r<10; r++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
c=Float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print ("Average is: "+d);
}
}
Joseph Andrew Batario
DIT 111
/**Create a program that will accept 10 floating point number.Compute and display the average**/
import java.io.*;
public class ASS1mjc
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader(System.in));
float m,j,c,ave;
m=0;j=0;ave=0;
for(j=0;j<10;j++)
{
System.out.print ("Enter number");
c=Float.parseFloat(x.readLine());
m=m+c;
}
ave=m/10;
System.out.print("Average is" +ave);
}
}
Michelle Calapit DIT111...
create a program that will accept 10 floating point number.Compute & display the average.
/*Rhodora M.Tagaya DIT111*/
gandang tanghali poh mam\/",)
e2 po ang comment q
import java.io.*;
public class ass1rmt
{
public static void main (String args []) throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
float m,r,t,e;
m=0;
r=0;
t=0;
for (r=0; r<10; r++)
{
System.out.print ("Enter number: ");
m=Float.parseFloat(x.readLine());
m=m+r;
}
e=m/10;
System.out.print ("Average is: "+e);
}
}
/*Create a program that will accept 10 floating point number.Compute and display the average.Ira Bernadette Jastiva DIT111*/ tama poh ba mam???
import java.io.*;
public class ass1ibj
{
public static void main (String args []) throws IOException
{
float i,b,j,p;
i=0; j=0; p=0;
for (b=0; b<10; b++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
i=Float.parseFloat(x.readLine());
i=i+j;}
p=i/10;
System.out.print ("Average is: "+p);
}
}
Problem:
Create a program that will accept 10 floating point numbers, compute and display the average. class name ASS1xxx.
import java.io.*;
public class ASS1rto
{
public static void main (String args []) throws IOException
{
float r,o,t,s;
r=0;
t=0;
s=0;
for (o=0; o<10; o++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
t=Float.parseFloat(x.readLine());
r=r+t;
}
s=r/10;
System.out.print ("Average is: "+s);
}
}
Rochelle Ocan
DIT111
Joseph Amparo
DIT111
Create a program that will accept 10 floating numbers. Compute and display the average. Class name ass1xxx.
import java.io.*;
public class ass1jca
{
public static void main (String args []) throws IOException
{
float j,a,s,w;
j=0;
s=0;
w=0;
for (a=0; a<10; a++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
s=Float.parseFloat(x.readLine());
j=j+s;
}
w=j/10;
System.out.print ("Average is: "+w);
}
}
import java.io.*;
public class ASS1csr
{
public static void main(String args[])throws IOException
{
float a,b,c,d;
a=b;
c=b;
d=b;
for(b=0;b<10;b++)
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter number:");
c=float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print("Average is:"+d);
}
}
Crestita S.Ramo
DIT111
October 8,2008/3:30pm
PROBLEM:Create a program that will accept 10 floating numbers, compute and display the average. Class name is Ass1xxx.
import java.io.*;
public class ASS1cmd
{
public static void main (String argas[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a,b,c,d;
b=0
c=b/10;
int num []= new int[10];
for(a=0;a<10;a++)
{
System.out.print("Enter number: \n" + (a+1)+">>");
num[a]=Integer.parseInt(x.readLine());
}
System.out.print("\n The Inputted number are:\n");
for (a=0;a<10;a++)
{System.out.print(""+num[a]);}
c=b/10;
{System.out.print("The average is"+b);}
}
}
Cecil M. Dalawampo
DIT111
PROBLEM:Create a program that will accept 10 floating numbers, compute and display the average. Class name is Ass1xxx.
import java.io.*;
public class ASS1cmd
{
public static void main (String argas[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a,b,c,d;
b=0
c=b/10;
int num []= new int[10];
for(a=0;a<10;a++)
{
System.out.print("Enter number: \n" + (a+1)+">>");
num[a]=Integer.parseInt(x.readLine());
}
System.out.print("\n The Inputted number are:\n");
for (a=0;a<10;a++)
{System.out.print(""+num[a]);}
c=b/10;
{System.out.print("The average is"+b);}
}
}
CECIL M. DALAWAMPO
DIT111
PROBLEM:Create a program that will accept 10 floating point numbers.Compute and display the average.Class name ASS1lal.
import java.io.*;
public class ASS1lal
{
public static void main(String args[])throws IOException
{
float a,b,c,d;
a=0;
b=0;
d=0;
for (b=0;b<10;b++)
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter number: ");
c=Float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print("Average is:"+d);
}
}
Lovely A.Leynes
DIT-111
sna po mam tma.....ang hrap po tlga eh,,,
PROBLEM:Create a program that will accept 10 floating point numbers.Compute and display the average.Class name ASS1lal.
import java.io.*;
public class ASS1lal
{
public static void main(String args[])throws IOException
{
float a,b,c,d;
a=0;
b=0;
d=0;
for (b=0;b<10;b++)
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter number: ");
c=Float.parseFloat(x.readLine());
a=a+c;
}
d=a/10;
System.out.print("Average is:"+d);
}
}
Lovely A.Leynes
DIT-111
sna po mam tma.....ang hrap po tlga eh,,,
PROBLEM:Create a program that will accept 10 floating point numbers.Compute and display the average.Class name ASS1xxx
import java.io.*;
public class ass1JED
{
public static void main (String args []) throws IOException
{
float e,m,e1,r;
e=0;
e1=0;
r=0;
for (m=0; m<10; m++)
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter number: ");
e1=Float.parseFloat(x.readLine());
e=e+e1;
}
r=e/10;
System.out.print ("Average is: "+r);
}
}
sana tumama 2.. .hirap kasi
kamoteness aq d2.. .
Emerson Dejoras DIT-111
import java.io.*;
public class ASS1AMA
{
public static void main (String argas[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int a,b,c,d;
b=0
c=b/10;
int num []= new int[10];
for(a=0;a<10;a++)
{
System.out.print("Enter number: \n" + (a+1)+">>");
num[a]=Integer.parseInt(x.readLine());
}
System.out.print("\n The Inputted number are:\n");
for (a=0;a<10;a++)
{System.out.print(""+num[a]);}
c=b/10;
{System.out.print("The average is"+b);}
}
}
hirap mam..........
Anthony Axalan Dit-111
Gagawin ko pa
Post ko nalang Mamaya!!
Mga 10pm po!
maam di pwedeng float ung array???
hmmm.. .pinuntahan q kc website ng java,ung bang
http://java.sun.com/docs/books/jls/second_edition/html/arrays.doc.html cguro un.. .puntahan nyu mam un at wala me makita.. .pki turo na lang po samen kung pwede ang array na may float kc d aq magkaige.. .hehehe
wla me mkita float eh
EMErson Dejoras DIT-111
play hard,Study harder
http://java.sun.com/docs/books/jls/second_edition/html/arrays.doc.html
puntahan nyu mam.. .
Mam ung iba tama kaso d gumamit na array!!!
PROBLEM:Create a program that will accept 10 floating numbers, compute and display the average. Class name is Ass1ssa.
import java.io.*;
public class ASS1ssa
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int s,t,u,v;
t=0;
u=t/10
int num[]=newint[10];
for(s=0;s<10;s++)
{
System.out.print("Enter number"+(s+1)+>>");
num[s]=Integer.parseInt(x.readLine());
}
System.out.print("\n\n The inputted numbers are:\n");
fot(s=0;s<10;s++)
{
System.out.println(""+num[s]);
}
}
}
Lovely A.Leynes
DIT-111
import java.io.*;
public class ASS1LHP
{
public static void main (String args []) throws IOException
{
BufferedReader leif=new BufferedReader (new InputStreamReader (System.in));
int l;
float num [] = new float[10],sum=0;
for ( l=0; l<10; l++)
{
System.out.print ("Enter number " + (l+1) + ": ");
num[l] = Float.parseFloat(leif.readLine());
sum=sum+num[l];
}
System.out.println("" +sum/10);
}
}
Yes Nakuha ko rin!!!!
Create a program that will accept 10 floating numbers. Compute and display the average. Class name is ASS1SSA.
import java.io.*;
public class ASS1lal
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
int;l,m,n,o;
int num[]=newint[10];
for(l=0;l<10;l++)
{
System.out.print("Enter number"+(l+1)+">>");
num[l]=Integer.parseInt(x.readLine());
}
System.out.print("\n\n The inputted numbers are:\n");
for(l=0;l<10;l++)
{
System.out.println(""+num[l];
System.out.print("Average is"+m");
}
}
}
Sherriel S. Azcarrate
DIT-111
PROBLEM:Create a program that will accept 10 floating numbers, compute and display the average. Class name is Ass1ssa.
import java.io.*;
public class ASS1csr
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader(new InputStreamReader(System.in));
float c[] = new float[10],s=0,l;
int r;
for(r=0;r<10;r++)
{
System.out.print("Enter number: ");
c[r]=Float.parseFloat(x.readLine());
s=s+c[r];
}
l=s/10;
System.out.print("Average is: "+l);
}
}
Crestita S. Ramo
DIT111
Mam sana tama....
import java.io.*;
public class ArrayBSIT112mtcb
{
public static void main (String args []) throws IOException
{
BufferedReader in=new BufferedReader (new InputStreamReader (System.in));
int a, ave,Math.sqrt, sum , x=10;
int num[];
num=new in [10];
for (a=0;a<10;a++)
{
System.out.print(("number:");
num[a]=Integer.parseInt(in.readLine());
}
sum=sum+num[a];
average=(sum)/x;
System.out.print(""+average);
for (a=0;a<10;a++)
if (average<75)
{
System.out.print(""+average);
}
for (a=0;a<10;a++)
else if (average>75)
{
System.out.print(intnum[a] + " " + Math.sqrt(intnum[a]));
}
for (a=0;a<10;a++)
else
{
System.out.print (" "+ sum);
}
}
}
PS:
AndaYa nYo nMn mam eh
nD nYo nMn tinUro kUng aNo kUnin sqrt.
/*WAP that will accept 20 integer number in an array. Determine and display all the even number. Display also the first and the last element of the array. Claas name ArrayDIT111rmt.
import java.io.*;
public class ArrayDIT111rmt
{
public static void main(String args[])throws IOException
{
BufferedReader j=new BufferedReader(new InputStreamReader(System.in));
int a,d=0;
int num[]=new int[20];
for(a=0;a<20;a++)
{
System.out.print("Enter number ["+(a+1)+"]: ");
num[a]=Integer.parseInt(j.readLine());
}
System.out.print("\n\n The even numbers are :\n");
for (a=0;a<20;a++)
{
d=num[a]%2;
if(d==0)
{
System.out.println(""+num[a]);
}
}
System.out.print("\n The 1st element of an array is "+num[0]);
System.out.print("\n The last element of an array is "+num[19]);
}
}
Andrian Caponpon
DIT 113
//*Try to create a program that will display the sum of 5 numbers in one dimensional of array
import java.io.*;
public class array1abc
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int s=0,a=0;
int abc[]=new int[5];
do
{System.out.print("Enter A Number: ");
abc[a]=Integer.parseInt(x.readLine());
a++;}
while(a<5);
s = abc[0] + abc[1]+ abc[2]+ abc[3]+ abc[4];
System.out.print("\nThe Sum is the Numbers is : "+s);
}
}
mam nagpaturo po ako jan sana tama.
Mark Anthony Flores
DIT 113
Second Comment
Agen
agen
making sure lang mam....
here is the ans.
import java.io.*;
public class array1mrf
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int sum=0,z=0;
int mrf[]=new int[6];
do
{System.out.print("Enter A Number: ");
mrf[z]=Integer.parseInt(x.readLine());
z++;}
while(z<5);
sum = mrf[0]+mrf[1]+mrf[2]+mrf[3]+mrf[4];
System.out.print("\nThe Sum is the Numbers is : "+sum);
}
}
Christian Z. Abastillas
DIT 113
//Try to create a program that will display the sum of 5 numbers in one dimensional of array//
import java.io.*;
public class ARRAY1CZA
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int f=0,d=0;
int CZA[]=new int[5];
do
{System.out.print("Enter A Number: ");
CZA[d]=Integer.parseInt(x.readLine());
d++;}
while(d<5);
f = CZA[0] + CZA[1]+ CZA[2]+ CZA[3]+ CZA[4];
System.out.print("\nThe Sum is the Numbers is : "+f);
}
}
yan po mam comment ko.....
Navarro R. Rofiles
DIT-113
//Try to create a program that will display the sum of 5 numbers in one dimensional of array//
import java.io.*;
public class ARRAY1NRR
{
public static void main(String args[])throws IOException
{
BufferedReader x=new BufferedReader (new InputStreamReader (System.in));
int rOn=0,p=0;
int NRR[]=new int[5];
do
{System.out.print("Enter A Integer Number: ");
NRR[p]=Integer.parseInt(x.readLine());
p++;}
while(p<5);
rOn=NRR[0]+NRR[1]+NRR[2]+NRR[3]+NRR[4];
System.out.print("\nThe Sum is the Numbers is : "+rOn);
}
}
____mam sana tama po ang ginawa ko...._____
Navarro R. Rofiles
DIT-113
/*
Create a program that will accept 20 prices of an item and store it in an array. Compute and
display the sum of all prices. Display all the values less that 5.00. Calculate and display
the average of the prices, and display all values higher than the calculated average value.
Class name is ArrayDIT113xxx.*/
import java.io.*;
public class ArrayDIT113jcs
{
public static void main (String args[])throws IOException
{
for(a=0;a<20;a++)
{
BufferedReader x=new BufferedReader (new InputStreamReader(System.in));
int a,b=0,c=0,s=0,ave1=0,ave2=0;
int num[]=new int[20];
for(a=0;a<5;a++)
{
System.out.print("Enter item price:");
a=Integer.parseInt(x.readLine());
s=s+price[a];
}
if (num[a]<20)
{
b=a+b;
ave1 =less/num[a];
}
else if(num[a]>20)
{
c=num[a] +high;
ave2 =high/num[a];
}
}
System.out.println("The sum of the 20 prices is " +sum);
System.out.println("The sum of the prices Less Than 5 is : " +less);
System.out.println("the average of the prices less than 5 is : " +ave1);
System.out.println("the sum of all prices higher than 5 is : " +high);
System.out.println("The average of all prices higher than 5 is : " +ave2);
}
}
mam ito po sagot ko julius salcedo po ito. DIT 113
import java.io.*;
public class BSIT112mtcb
{
public static void main (String args []) throws IOException
{
BufferedReader in=new BufferedReader (new InputStreamReader (System.in));
int a, sum=0, ave=0;
int num = new int[10];
System.out.print("Enter number:");
num[a]=Integer.parseInt(in.readLine());
sum=sum+num[a];
ave=(sum)/10;
for(a=0,a<10,a++)
if (a<75)
{
System.out.print(""+ave);
}
for(a=0,a<10,a++)
if (a>75)
{
System.out.print(""+Math.sqrt(ave));
}
else
System.out.print(""+sum);
}
}
import java.io.*;
public class BSIT112mtcb
{
public static void main (String args []) throws IOException
{
BufferedReader in=new BufferedReader (new InputStreamReader (System.in));
int a, sum=0, ave=0;
int num = new int[10];
System.out.print("Enter number:");
num[a]=Integer.parseInt(in.readLine());
sum=sum+num[a];
ave=(sum)/10;
for(a=0,a<10,a++)
if (a<75)
{
System.out.print(""+ave);
}
for(a=0,a<10,a++)
if (a>75)
{
System.out.print(""+Math.sqrt(ave));
}
else
System.out.print(""+sum);
}
}
//* LADY JOY M. QUERIJERO *//
//* DIT 112 *//
import java.io.*;
public class ArrayDIT112LMQ
{
public static void main(String[]args)throws IOException
{
BufferedREder joy=new BufferedReader(new Input StreamReader(System.in));
int L;
int num []=new int [5];
for (L=0; L<5; L++)
{
System.out.print("Enter first to last number" +"~~~");
num[L]= Integer.parseInt(x.readLine());
}
System.out.print("num+[L]");
}
}
hehehehe mam.... hula lng po...~~~ mam sakit na ulo ko... mam pno po b gwin yun? D ko po kc mplbs yung pinapa sagutan niyo po...>>>ahaaiii
lady joy querijero
import java.io.*;
public class Arraydit112LMQ
{
public static void main(String[] args)throws IOException
{
BufferedReader joy=new BufferedReader(new InputStreamReader(System.in));
int L;
int num[]= new new[5];
for (L=0; L<5; L++)
{
System.out.print("Enter first to last number" +"~~~");
num[L]= Integer.parseInt("joy.readLine());
}
System.out.print("\n display the number:\n");
for (L=0; L<5; L++)
{
System.out.print("+num[L]");
}
}
hehehe mam mali yung isa.....ahaaaiii>>>>
09106948import java.io.*;
public class ArrayDIT111KAQ
{
public static void main(String args[])throws IOException
{
BufferedReader kristine=new BufferedReader(new InputStreamReader(System.in));
int a,d=0;
int num[]=new int[20];
for(a=0;a<20;a++)
{
System.out.print("Enter number "+(a+1)+": ");
num[a]=Integer.parseInt(kristine.readLine());
}
System.out.print("\n\n The even numbers are :\n");
for (a=0;a<20;a++)
{
d=num[a]%2;
if(d==0)
{
System.out.println(""+num[a]);
}
}
System.out.print("\n The 1st element of an array is "+num[0]);
System.out.print("\n The last element of an array is "+num[19]);
}
}
DIT- Kristine Quiling..^_^
Post a Comment