A Java applet is such a program written in the Java programming language and typically designed to run from a web browser.
Creating a Java Applet
1 . Write the applet in Java, and save it with a .java file extension, just as when you write a Java application.
2. Compile the applet into bytecode using the javac command, just as when you write a Java application.
3. Write an HTML document that includes a statement to call your compiled Java class.
4. Load the HTML document into a Web browser (such as Mozilla Firefox or Microsoft Internet Explorer), or open the HTML document using the Applet Viewer.
Creating HTML document
1. The tag that begins every HTML document is , which is surrounded by angle brackets.
2. The html within the tag is an HTML keyword that specifies that an HTML document follows the keyword.
3. The tag that ends every HTML document is .
Generally, HTML documents contain other tags with the texts. Of importance at this point is the tag pair used to run an applet from within an HTML document.
Three attributes (or arguments) are placed within the
Three attributes of the given object tag:
1. code = followed by the name of the compiled applet you are calling
2. width = followed by the width of the applet on the screen
3. height = followed by the height of the applet on the screen
Running an Applet
Two ways of running an applet:
1. by opening the associated HTML file on a Web browser
2. the appletviewer
Writing a Simple Applet
In creating an applet, you must also do the following:
1. Include import statements to ensure that necessary classes are available.
2. Learn to use some user interface (UI) components, such as buttons and text fields, and applet methods.
3. Learn to use the keyword extends.
Creators of Java created an applet class named JApplet that you can import using the statement,
import javax.swing.JApplet;
JApplet Class
JApplet is the Swing equivalent of the AWT Applet class.It is a simple extension of java.applet.Applet for use when creating Swing programs designed to be used in a Web browser.
As a direct subclass of Applet, JApplet is used in much the same way as the Applet.
A JApplet is a Component, and it is also a Container.
When using the JApplet class, you need the following import statements:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
The extends keyword indicates that your applet builds on, or inherits, the traits of the JApplet class.
Example 1:
//java file... save as .java file
import javax.swing.*;
import java.awt.*;
public class sampleApplet extends JApplet
{
public void init()
{
Container con = getContentPane();
cont.setLayout(new FlowLayout());
JLabel label = new JLabel("Hello world!");
con.add(label);
}
}
------------------------------
// html file... save as .html file
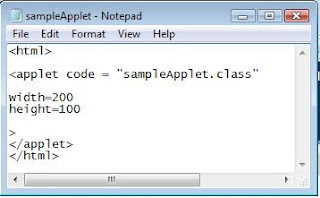
Output:
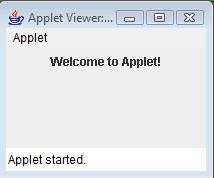
//java file... save as .java file
import javax.swing.*;
import java.awt.event.*;
import java.awt.*;
public class sampleAppletRRA extends JApplet implements ActionListener
{
Container con = getContentPane();
JLabel question = new JLabel("What’s your name?");
JTextField answer = new JTextField(10);
JButton press = new JButton("Press me");
public void init()
{
con.add(question);
con.add(answer);
con.add(press);
con.setLayout(new FlowLayout());
press.addActionListener(this);
}
public void actionPerformed(ActionEvent e)
{
String name = answer.getText();
JOptionPane.showMessageDialog(null, "You pressed the button, " + name);
}
}
------------------------------
//html file... save as .html file
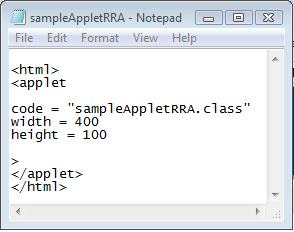
Output:
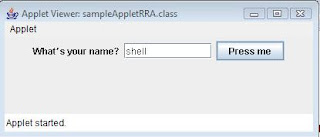
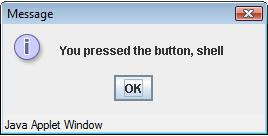
Try to create an applet that will accept 2 integer numbers and display the sum.
27 comments:
venus sun dit211
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Appletq extends Applet implements ActionListener {
Button btn1, btn2;
Label lbl1,lbl2;
TextField txt1,txt2;
public void init()
{
setVisible(true);
setSize(300,250);
setLayout(null);
btn1=new Button("SUM");
btn1.setBounds(15,140,90,40);
btn1.addActionListener(this);
add(btn1);
btn2=new Button("CLEAR");
btn2.setBounds(150,140,90,40);
btn2.addActionListener(this);
add(btn2);
lbl1=new Label("Number Here!");
lbl1.setBounds(40,50,80,40);
add(lbl1);
lbl2=new Label("Number Again!");
lbl2.setBounds(40,90,90,40);
add(lbl2);
txt1=new TextField("");
txt1.setBounds(170,50,80,40);
add(txt1);
txt2=new TextField("");
txt2.setBounds(170,90,80,40);
add(txt2);
}
public void actionPerformed(ActionEvent h)
{
if(h.getSource()==btn1)
{
int a,b,c;
a=Integer.parseInt(txt1.getText());
b=Integer.parseInt(txt2.getText());
c=a+b;
JOptionPane.showMessageDialog(null,"Answer is " +c);
}
if(h.getSource()==btn2)
{
txt1.setText("");
txt2.setText("");
btn1.setEnabled(true);
}
}
}
//By LEO "POGI" SANGALANG
//DIT 211 STI-LUCENA CITY
//SSS# 02876622
//txt me at 09095146361
import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class LEOAPPLET extends Applet implements ActionListener
{
Button sum,clear;
Label lbl1,lbl2,lbl3;
TextField txt1, txt2;
public void init()
{
setVisible(true);
setSize(225,220);
setLayout(null);
setBackground(Color.black);
Font pogi = new Font("arial",Font.BOLD,13);
sum = new Button("SUM");
sum.setBounds(10,140,80,30);
sum.addActionListener(this);
sum.setForeground(Color.blue);
sum.setBackground(Color.black);
sum.setFont(pogi);
add(sum);
clear = new Button("CLEAR");
clear.setBounds(95,140,120,30);
clear.addActionListener(this);
clear.setForeground(Color.orange);
clear.setBackground(Color.black);
clear.setFont(pogi);
add(clear);
lbl1=new Label("Enter a Number");
lbl1.setBounds(10,50,80,40);
lbl1.setForeground(Color.red);
lbl1.setFont(pogi);
add(lbl1);
lbl2=new Label("2nd Number");
lbl2.setBounds(10,90,80,40);
lbl2.setForeground(Color.red);
lbl2.setFont(pogi);
add(lbl2);
lbl3=new Label("");
lbl3.setBounds(100,170,120,40);
lbl3.setForeground(Color.red);
lbl3.setFont(pogi);
Font pogi2 = new Font("arial",Font.BOLD,40);
lbl3.setFont(pogi2);
add(lbl3);
txt1=new TextField("");
txt1.setBounds(90,50,125,25);
txt1.setFont(pogi);
add(txt1);
txt2=new TextField("");
txt2.setBounds(90,90,125,25);
txt2.setFont(pogi);
add(txt2);
}
public void actionPerformed(ActionEvent LEOPOGI)
{
if(LEOPOGI.getSource()==sum)
{
int a,b,summ;
a = Integer.parseInt(txt1.getText());
b = Integer.parseInt(txt2.getText());
summ = a+b;
lbl3.setText(""+summ);
}
if(LEOPOGI.getSource()==clear)
{
txt1.setText("");
txt2.setText("");
lbl3.setText("");
}
}
}
http://img518.imageshack.us/my.php?image=leopogiwm0.jpg
NOYMIE MARTILLANO
DIT211
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class PFQUIZ2 extends Applet implements ActionListener
{
Button sum,clear;
Label lblnum1,lblnum2,lbl3;
TextField txtnum1,txtnum2;
public void init()
{
setVisible(true);
setSize(320,350);
setLayout(null);
setBackground(Color.yellow);
sum=new Button("SUM");
sum.setBounds(180,200,60,40);
sum.addActionListener(this);
add(sum);
clear=new Button("CLEAR");
clear.setBounds(120,200,60,40);
clear.addActionListener(this);
add(clear);
lblnum1=new Label("1st Number");
lblnum1.setBounds(30,90,80,50);
lblnum1.setForeground(Color.blue);
add(lblnum1);
lblnum2=new Label("2nd Number");
lblnum2.setBounds(30,120,80,50);
lblnum2.setForeground(Color.blue);
add(lblnum2);
lbl3=new Label("");
lbl3.setBounds(120,160,60,30);
Font b=new Font("Tahoma",Font.BOLD,36);
lbl3.setFont(b);
add(lbl3);
txtnum1=new TextField("");
txtnum1.setBounds(120,90,60,30);
add(txtnum1);
txtnum2=new TextField("");
txtnum2.setBounds(120,125,60,30);
add(txtnum2);
}
public void actionPerformed(ActionEvent noemi)
{
if(noemi.getSource()==sum)
{
int num1,num2,ans;
num1=Integer.parseInt(txtnum1.getText());
num2=Integer.parseInt(txtnum2.getText());
ans=num1+num2;
lbl3.setText(""+ans);
}
if(noemi.getSource()==clear)
{
txtnum1.setText("");
txtnum2.setText("");
lbl3.setText("");
}
}
}
Nadine Cristine Anne Garcia
dit211
import java.applet.*;
import javax.swing.*;
import java.awt.event.*;
import java.awt.*;
public class sampleApplet2 extends Applet implements ActionListener
{
Button sum;
Label lbl1stnum,lbl2ndnum;
TextField txt1stnum,txt2ndnum;
public void init()
{
setVisible(true);
setSize(300,300);
setLayout(null);
sum=new Button("SUM");
sum.setBounds(40,250,80,40);
sum.addActionListener(this);
add(sum);
txt1stnum=new TextField("");
txt1stnum.setBounds(190,90,80,40);
add(txt1stnum);
txt2ndnum=new TextField("");
txt2ndnum.setBounds(190,50,80,40);
add(txt2ndnum);
lbl1stnum=new Label("nads");
lbl1stnum.setBounds(30,50,80,40);
add(lbl1stnum);
lbl2ndnum=new Label("nads");
lbl2ndnum.setBounds(30,90,90,40);
add(lbl2ndnum);
}
public void actionPerformed(ActionEvent nads)
{
if(nads.getSource()==sum)
{
int fstnum,sndnum,ans;
fstnum=Integer.parseInt(txt1stnum.getText());
sndnum=Integer.parseInt(txt2ndnum.getText());
ans=fstnum+sndnum;
JOptionPane.showMessageDialog(null,"the sum is" + ans);
}
}
}
http://img518.imageshack.us/my.php?image=leopogiwm0.jpg
[URL=http://img518.imageshack.us/my.php?image=leopogiwm0.jpg][IMG]http://img518.imageshack.us/img518/8828/leopogiwm0.th.jpg[/IMG][/URL]
http://img518.imageshack.us/img518/8828/leopogiwm0.jpg
pls click for LEO POGI
Tyson Anlacan
BSCoE 411
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class AppletTMA extends Applet implements ActionListener {
Button comp, clr;
Label lblnum1, lblnum2, lblsum;
TextField txtnum1, txtnum2, txtsum;
public static void main(String args[]){
AppletTMA s=new AppletTMA ();
s.addWindowListener(new WindowAdapter()
{public void windowClosing(WindowEvent a)
{System.exit(0);}});
}
AppletTMA (){
setVisible(true);
setTitle("Applet");
setSize(260,400);
setLayout(null);
setResizable(false);
setBackground(Color.black);
lblnum1=new Label("Enter 1st number: ");
lblnum1.setBounds(20,50,110,30);
lblnum1.setBackground(Color.black);
lblnum1.setForeground(Color.green);
add(lblnum1);
lblnum2= new Label("Enter 2nd number: ");
lblnum2.setBounds(20,100,110,30);
lblnum2.setBackground(Color.black);
lblnum2.setForeground(Color.green);
add(lblnum2);
lblsum= new Label("Enter 3rd number: ");
lblsum.setBounds(20,150,110,30);
lblsum.setBackground(Color.black);
lblsum 3.setForeground(Color.green);
add(lblsum);
txtnum1=new TextField("");
txtnum1.setBounds(140,50,80,30);
add(txtnum1);
txtnum2= new TextField("");
txtnum2.setBounds(140,100,80,30);
add(txtnum2);
txtsum= new TextField("");
txtsum.setBounds(140,150,80,30);
add(txtsum);
comp=new Button("COMPUTE");
comp setBounds(80,350,80,30);
add(comp);
comp.addActionListener(this);
clr=new Button("CLEAR");
clr.setBounds(80,350,80,30);
add(clr);
clr.addActionListener(this);
}
public void actionPerformed(ActionEvent sm){
int num1,num2,ans=0;
num1=Integer.parseInt(txtnum1.getText());
num2=Integer.parseInt(txtnum2.getText());
if(sm.getSource()==comp){
ans=num1+num2;
txtsum.setText(""+ans);
JOptionPane.showMessageDialog(null,"The answer is " +ans);
if(sm.getSource()==clr){
txtnum1.setText("");
txtnum2.setText("");
txtsum.setText("");
}
}
}
Jeyfren Manalo
BSCoE 4-1-1
ASSIGNMENT
import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class JEYFAPPLET extends Applet implements ActionListener
{
Button sum,clear;
Label lbl1,lbl2,lbl3;
TextField txt1, txt2;
public void init()
{
setVisible(true);
setSize(300,250);
setLayout(null);
setBackground(Color.black);
Font jey = new Font("arial",Font.BOLD,13);
sum = new Button("SUM");
sum.setBounds(10,140,80,30);
sum.addActionListener(this);
sum.setForeground(Color.blue);
sum.setBackground(Color.black);
sum.setFont(jey);
add(sum);
clear = new Button("CLEAR");
clear.setBounds(140,140,120,30);
clear.addActionListener(this);
clear.setForeground(Color.orange);
clear.setBackground(Color.black);
clear.setFont(jey);
add(clear);
lbl1=new Label("Enter 1st Number: ");
lbl1.setBounds(10,50,120,40);
lbl1.setForeground(Color.red);
lbl1.setFont(jey);
add(lbl1);
lbl2=new Label("Enter 2nd Number: ");
lbl2.setBounds(10,90,120,40);
lbl2.setForeground(Color.red);
lbl2.setFont(jey);
add(lbl2);
lbl3=new Label("");
lbl3.setBounds(100,180,120,40);
lbl3.setForeground(Color.red);
lbl3.setFont(jey);
Font jey2 = new Font("arial",Font.BOLD,40);
lbl3.setFont(jey2);
add(lbl3);
txt1=new TextField("");
txt1.setBounds(140,50,125,25);
txt1.setFont(jey);
add(txt1);
txt2=new TextField("");
txt2.setBounds(140,90,125,25);
txt2.setFont(jey);
add(txt2);
}
public void actionPerformed(ActionEvent J)
{
if(J.getSource()==sum)
{
int a,b,sm;
a = Integer.parseInt(txt1.getText());
b = Integer.parseInt(txt2.getText());
sm = a+b;
lbl3.setText(""+sm);
}
if(J.getSource()==clear)
{
txt1.setText("");
txt2.setText("");
lbl3.setText("");
}
}
}
emmanuel correa
BSCoE
import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class EMZAPPLET extends Applet implements ActionListener
{
Button sum,clear;
Label lbl1,lbl2,lbl3;
TextField txt1, txt2;
public void init()
{
setVisible(true);
setSize(300,250);
setLayout(null);
setBackground(Color.red);
Font emman = new Font("arial",Font.BOLD,13);
sum = new Button("SUM");
sum.setBounds(10,140,80,30);
sum.addActionListener(this);
sum.setForeground(Color.green);
sum.setBackground(Color.black);
sum.setFont(emman);
add(sum);
clear = new Button("CLEAR");
clear.setBounds(140,140,120,30);
clear.addActionListener(this);
clear.setForeground(Color.orange);
clear.setBackground(Color.red);
clear.setFont(emman);
add(clear);
lbl1=new Label("1st Number");
lbl1.setBounds(10,50,80,40);
lbl1.setForeground(Color.red);
lbl1.setFont(emman);
add(lbl1);
lbl2=new Label("2nd Number");
lbl2.setBounds(10,90,80,40);
lbl2.setForeground(Color.red);
lbl2.setFont(emman);
add(lbl2);
lbl3=new Label("");
lbl3.setBounds(100,170,120,40);
lbl3.setForeground(Color.red);
lbl3.setFont(emman);
Font pogi2 = new Font("arial",Font.BOLD,40);
lbl3.setFont(emman2);
add(lbl3);
txt1=new TextField("");
txt1.setBounds(90,50,125,25);
txt1.setFont(emman);
add(txt1);
txt2=new TextField("");
txt2.setBounds(90,90,125,25);
txt2.setFont(emman);
add(txt2);
}
public void actionPerformed(ActionEvent EMMANZ)
{
if(EMMANZ.getSource()==sum)
{
int a,b,sum;
a = Integer.parseInt(txt1.getText());
b = Integer.parseInt(txt2.getText());
sum = a+b;
lbl3.setText(""+sum);
}
if(EMMANZ.getSource()==clear)
{
txt1.setText("");
txt2.setText("");
lbl3.setText("");
}
}
}
Benedict PeƱaflor
BSCoE 4-1/1
Assignment in JAVA
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class BGPAPPLET extends Applet implements ActionListener
{
Button sum,clear;
Label lblnum1,lblnum2,lbl3;
TextField txtnum1,txtnum2;
public void init()
{
setVisible(true);
setSize(320,350);
setLayout(null);
setBackground(Color.black);
sum=new Button("SUM");
sum.setBounds(180,200,60,40);
sum.addActionListener(this);
add(sum);
clear=new Button("CLEAR");
clear.setBounds(120,200,60,40);
clear.addActionListener(this);
add(clear);
lblnum1=new Label("1st Number");
lblnum1.setBounds(30,90,80,50);
lblnum1.setForeground(Color.blue);
add(lblnum1);
lblnum2=new Label("2nd Number");
lblnum2.setBounds(30,120,80,50);
lblnum2.setForeground(Color.blue);
add(lblnum2);
lbl3=new Label("");
lbl3.setBounds(120,160,60,30);
Font b=new Font("Tahoma",Font.BOLD,36);
lbl3.setFont(b);
add(lbl3);
txtnum1=new TextField("");
txtnum1.setBounds(120,90,60,30);
add(txtnum1);
txtnum2=new TextField("");
txtnum2.setBounds(120,125,60,30);
add(txtnum2);
}
public void actionPerformed(ActionEvent dick)
{
if(dick.getSource()==sum)
{
int num1,num2,ans;
num1=Integer.parseInt(txtnum1.getText());
num2=Integer.parseInt(txtnum2.getText());
ans=num1+num2;
lbl3.setText(""+ans);
}
if(dick.getSource()==clear)
{
txtnum1.setText("");
txtnum2.setText("");
lbl3.setText("");
}
}
}
John Ryan Quijano
BSCoE 4-1/1
Assignment in JAVA
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class RYANQ extends Applet implements ActionListener
{
Button sum,clear;
Label lbl1,lbl2,lbl3;
TextField txt1,txt2;
public void init()
{
setVisible(true);
setSize(320,350);
setLayout(null);
setBackground(Color.black);
sum=new Button("SUM");
sum.setBounds(180,200,60,40);
sum.addActionListener(this);
add(sum);
clear=new Button("CLEAR");
clear.setBounds(120,200,60,40);
clear.addActionListener(this);
add(clear);
lbl1=new Label("1st Number");
lbl1.setBounds(30,90,80,50);
lbl1.setForeground(Color.blue);
add(lbl1);
lbl2=new Label("2nd Number");
lbl2.setBounds(30,120,80,50);
lbl2.setForeground(Color.blue);
add(lbl2);
lbl3=new Label("");
lbl3.setBounds(120,160,60,30);
Font b=new Font("Tahoma",Font.BOLD,36);
lbl3.setFont(b);
add(lbl3);
txt1=new TextField("");
txt1.setBounds(120,90,60,30);
add(txt1);
txt2=new TextField("");
txt2.setBounds(120,125,60,30);
add(txt2);
}
public void actionPerformed(ActionEvent ryan)
{
if(ryan.getSource()==sum)
{
int num1,num2,ans;
num1=Integer.parseInt(txt1.getText());
num2=Integer.parseInt(txt2.getText());
ans=num1+num2;
lbl3.setText(""+ans);
}
if(ryan.getSource()==clear)
{
txt1.setText("");
txt2.setText("");
lbl3.setText("");
}
}
}
jay jaber ramirez
BSCoE
import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class JABAPPLET extends Applet implements ActionListener
{
Button sum,clear;
Label lbl1,lbl2,lbl3;
TextField txt1, txt2;
public void init()
{
setVisible(true);
setSize(300,250);
setLayout(null);
setBackground(Color.red);
Font jaber = new Font("arial",Font.BOLD,13);
sum = new Button("SUM");
sum.setBounds(10,140,80,30);
sum.addActionListener(this);
sum.setForeground(Color.green);
sum.setBackground(Color.black);
sum.setFont(jaber);
add(sum);
clear = new Button("CLEAR");
clear.setBounds(140,140,120,30);
clear.addActionListener(this);
clear.setForeground(Color.orange);
clear.setBackground(Color.red);
clear.setFont(jaber);
add(clear);
lbl1=new Label("A 1st Number");
lbl1.setBounds(10,50,80,40);
lbl1.setForeground(Color.red);
lbl1.setFont(jaber);
add(lbl1);
lbl2=new Label("A 2nd Number");
lbl2.setBounds(10,90,80,40);
lbl2.setForeground(Color.red);
lbl2.setFont(jaber);
add(lbl2);
lbl3=new Label("");
lbl3.setBounds(100,170,120,40);
lbl3.setForeground(Color.red);
lbl3.setFont(jaber);
Font jaber2 = new Font("arial",Font.BOLD,40);
lbl3.setFont(jaber2);
add(lbl3);
txt1=new TextField("");
txt1.setBounds(90,50,125,25);
txt1.setFont(jaber);
add(txt1);
txt2=new TextField("");
txt2.setBounds(90,90,125,25);
txt2.setFont(jaber);
add(txt2);
}
public void actionPerformed(ActionEvent JABER)
{
if(JABER.getSource()==sum)
{
int a,b,summ;
a = Integer.parseInt(txt1.getText());
b = Integer.parseInt(txt2.getText());
summ = a+b;
lbl3.setText(""+summ);
}
if(JABER.getSource()==clear)
{
txt1.setText("");
txt2.setText("");
lbl3.setText("");
}
}
}
henry ruz dit/221
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
public class appletHLR extends JApplet implements ActionListener
{
Container con = getContentPane();
JLabel question = new JLabel("who's the greatest?");
JTextField answer = new JTextField(10);
JButton press = new JButton("click me");
String name = "HENRY";
public void init()
{
con.add(question);
con.add(answer);
con.add(click me);
con.setLayout(new FlowLayout());
press.addActionListener(this);
}
public void actionPerformed(ActionEvent e)
{
String name = answer.getText();
JOptionPane.showMessageDialog(null, "who's the greates?" + name);
}
}
Write a program that will accept 10 integer numbers store in an array. Compute and display the average. If the average is below 75 display the average, if the average is above 75 compute and display the square root of the average otherwise display the sum. Class name is ArrayBSIT112xxx.
Hi Maam!!
Jerick I Bayotas
BSIT 112
import java.io.*;
public class ArrayBSIT112JIB
{
public static void main(String args[])throws IOException
BufferedReader x = new BufferedReader (new InputStreamReader (System.in));
int a, b, c, d, ave, sum;
sum=0;
int [];
num = new int[10];
for (a=0; b<10; c++)
{
System.out.print("Enter number " + (a+1) + "<^_^>");
num[a]=Integer.parseInt(x.readLine());
}
System.out.print("\n\n The numbers are: \n ");
ave = (b)/10;
sum = sum num[a];
if (a<75)
System.out.print("Input a number ");
a=Integer.parseInt(x.readLine());
System.out.print("\n ");
if (a>75)
System.out.print("Input a number ");
a=Integer.parseInt(x.readLine());
System.out.print("\n ");
else
System.out.print("The sum is " + sum);
}
}
Mam d poh aq sure s sgot koh!!
nagu2luhan aq eh!!!
sna nmn poh my tumama?
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class AppletHLR extends Applet implements ActionListener {
Button comp, clr;
Label lbl1, lbl2, lblsum;
TextField txt1, txt2, txtsum;
public static void main(String args[]){
AppletHLRs=new AppletHLR();
s.addWindowListener(new WindowAdapter()
{public void windowClosing(WindowEvent a)
{System.exit(0);}});
}
AppletHLR(){
setVisible(true);
setTitle("Applet");
setSize(260,400);
setLayout(null);
setResizable(false);
setBackground(Color.black);
lbl1=new Label("Enter 1st number: ");
lbl1.setBounds(20,50,110,30);
lbl1.setBackground(Color.black);
lbl1.setForeground(Color.green);
add(lbl1);
lbl2= new Label("Enter 2nd number: ");
lbl2.setBounds(20,100,110,30);
lbl2.setBackground(Color.black);
lbl2.setForeground(Color.green);
add(lbl2);
lblsum= new Label("Enter 3rd number: ");
lblsum.setBounds(20,150,110,30);
lblsum.setBackground(Color.black);
lblsum 3.setForeground(Color.green);
add(lblsum);
txt1=new TextField("");
txt1.setBounds(140,50,80,30);
add(txt1);
txt2= new TextField("");
txt2.setBounds(140,100,80,30);
add(txt2);
txtsum= new TextField("");
txtsum.setBounds(140,150,80,30);
add(txtsum);
comp=new Button("COMPUTE");
comp setBounds(80,350,80,30);
add(comp);
comp.addActionListener(this);
clr=new Button("CLEAR");
clr.setBounds(80,350,80,30);
add(clr);
clr.addActionListener(this);
}
public void actionPerformed(ActionEvent sm){
int a,b,ans=0;
a=Integer.parseInt(txtnum1.getText());
a=Integer.parseInt(txtnum2.getText());
if(sm.getSource()==comp){
ans=a+a;
txtsum.setText(""+ans);
JOptionPane.showMessageDialog(null,"The answer is " +ans);
if(sm.getSource()==clr){
txt1.setText("");
txt2.setText("");
txtsum.setText("");
}
}
}
MELNIE MALAPITAN
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class SUMAPPLET extends Applet implements ActionListener
{
Button sum,clear;
Label lbl1,lbl2;
TextField txt1,txt2;
public void init()
{
setVisible(true);
setSize(400,400);
setBackground(Color.PINK);
setLayout(null);
sum=new Button("SUM");
sum.setBackground(Color.RED);
sum.setBounds(15,140,90,40);
sum.addActionListener(this);
add(sum);
clear=new Button("CLEAR");
clear.setBackground(Color.RED);
clear.setBounds(15,200,90,40);
clear.addActionListener(this);
add(clear);
lbl1=new Label("1st NUMBER:");
lbl1.setBounds(40,50,80,40);
add(lbl1);
lbl2=new Label("2nd NUMBER:");
lbl2.setBounds(40,90,90,40);
add(lbl2);
txt1=new TextField("");
txt1.setBackground(Color.ORANGE);
txt1.setBounds(170,50,80,40);
add(txt1);
txt2=new TextField("");
txt2.setBackground(Color.ORANGE);
txt2.setBounds(170,90,80,40);
add(txt2);
}
public void actionPerformed(ActionEvent w)
{
if(w.getSource()==sum)
{
int a,b,c;
a=Integer.parseInt(txt1.getText());
b=Integer.parseInt(txt2.getText());
c=a+b;
JOptionPane.showMessageDialog(null,"Answer is " +c);
}
if(w.getSource()==clear)
{
txt1.setText("");
txt2.setText("");
sum.setEnabled(true);
clear.setEnabled(true);
}
}
}
Ronalyn Miral DIT 211
//import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class SUMDIT211 extends Applet implements ActionListener
{
Button sum,clear;
Label lbl1,lbl2,lbl3;
TextField txt1, txt2;
public void init()
{
setVisible(true);
setSize(225,220);
setLayout(null);
setBackground(Color.black);
Font pogi = new Font("arial",Font.BOLD,13);
sum = new Button("SUM");
sum.setBounds(10,140,80,30);
sum.addActionListener(this);
sum.setForeground(Color.blue);
sum.setBackground(Color.black);
sum.setFont(rhonna);
add(sum);
clear = new Button("CLEAR");
clear.setBounds(95,140,120,30);
clear.addActionListener(this);
clear.setForeground(Color.orange);
clear.setBackground(Color.black);
clear.setFont(rhonna);
add(clear);
lbl1=new Label("Enter a Number");
lbl1.setBounds(10,50,80,40);
lbl1.setForeground(Color.red);
lbl1.setFont(rhonna);
add(lbl1);
lbl2=new Label("2nd Number");
lbl2.setBounds(10,90,80,40);
lbl2.setForeground(Color.red);
lbl2.setFont(rhonna);
add(lbl2);
lbl3=new Label("");
lbl3.setBounds(100,170,120,40);
lbl3.setForeground(Color.red);
lbl3.setFont(rhonna);
Font pogi2 = new Font("arial",Font.BOLD,40);
lbl3.setFont(pogi2);
add(lbl3);
txt1=new TextField("");
txt1.setBounds(90,50,125,25);
txt1.setFont(rhonna);
add(txt1);
txt2=new TextField("");
txt2.setBounds(90,90,125,25);
txt2.setFont(rhonna);
add(txt2);
}
public void actionPerformed(ActionEvent RHONNALYN)
{
if(RHONNALYN.getSource()==sum)
{
int a,b,summ;
a = Integer.parseInt(txt1.getText());
b = Integer.parseInt(txt2.getText());
summ = a+b;
lbl3.setText(""+summ);
}
if(RHONNALYN.getSource()==clear)
{
txt1.setText("");
txt2.setText("");
lbl3.setText("");
}
}
}
jeralyn garay dit211... tnx po galing...grabe
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Applet extends Applet implements ActionListener {
Button btn1, btn2;
Label lbl1,lbl2;
TextField txt1,txt2;
public void init()
{
setVisible(true);
setSize(300,250);
setLayout(null);
btn1=new Button("SUM");
btn1.setBounds(20,145,95,45);
btn1.addActionListener(this);
add(btn1);
btn2=new Button("CLEAR");
btn2.setBounds(150,140,90,40);
btn2.addActionListener(this);
add(btn2);
lbl1=new Label("Number Here!");
lbl1.setBounds(40,55,85,40);
add(lbl1);
lbl2=new Label("Number Again!");
lbl2.setBounds(50,90,80,40);
add(lbl2);
txt1=new TextField("");
txt1.setBounds(170,50,80,40);
add(txt1);
txt2=new TextField("");
txt2.setBounds(170,90,80,40);
add(txt2);
}
public void actionPerformed(ActionEvent h)
{
if(h.getSource()==btn1)
{
int a,b,c;
a=Integer.parseInt(txt1.getText());
b=Integer.parseInt(txt2.getText());
c=a+b;
JOptionPane.showMessageDialog(null,"Answer is " +c);
}
if(h.getSource()==btn2)
{
txt1.setText("");
txt2.setText("");
btn1.setEnabled(true);
}
}
}
BY: LEO "pogi" SANGALANG
//Create an applet with a Button and TextField.
//Input your name and display it in an 8pt font when button is cliked.
//Everytime the user clicks the Button,
//increase the font size for the displayed name by four points.
//Remove the Button when the font size exceeds 24 points.
//Class name is AppletDIT211xxx.
import java.awt.event.*;
import java.awt.*;
import javax.swing.*;
import java.applet.*;
public class AppletDIT211LEO extends Applet implements ActionListener
{
Button b1,b2,b3,b4,b5,b6;
Label l1,l2;
TextField t1;
public void init()
{
setVisible(true);
setSize(300,200);
setLayout(null);
setBackground(Color.black);
b1 = new Button("Enter");
b1.setBounds(200,50,60,20);
b1.setForeground(Color.red);
b1.addActionListener(this);
add(b1);
b2 = new Button("Enter");
b2.setBounds(200,50,60,20);
b2.setForeground(Color.red);
b2.addActionListener(this);
b2.setVisible(false);
add(b2);
b3 = new Button("Enter");
b3.setBounds(200,50,60,20);
b3.setForeground(Color.red);
b3.addActionListener(this);
b3.setVisible(false);
add(b3);
b4 = new Button("Enter");
b4.setBounds(200,50,60,20);
b4.setForeground(Color.red);
b4.addActionListener(this);
b4.setVisible(false);
add(b4);
b5 = new Button("Enter");
b5.setBounds(200,50,60,20);
b5.setForeground(Color.red);
b5.addActionListener(this);
b5.setVisible(false);
add(b5);
b6 = new Button("Enter");
b6.setBounds(200,50,60,20);
b6.setForeground(Color.red);
b6.addActionListener(this);
b6.setVisible(false);
add(b6);
t1=new TextField("");
t1.setBounds(30,50,150,20);
add(t1);
l2 = new Label("you have gone over the font size limit!");
l2.setBounds(10,140,500,40);
l2.setForeground(Color.blue);
Font qw=new Font("arial",Font.BOLD,15);
l2.setFont(qw);
l2.setVisible(false);
add(l2);
l1=new Label("");
l1.setBounds(120,100,120,30);
l1.setForeground(Color.red);
Font a=new Font("arial",Font.ITALIC,8);
l1.setFont(a);
add(l1);
}
public void actionPerformed(ActionEvent leopogi)
{
if(leopogi.getSource()==b1)
{
Font m = new Font("arial",Font.BOLD,8);
l1.setText(t1.getText());
l1.setFont(m);
b1.setVisible(false);
b2.setVisible(true);
}
if(leopogi.getSource()==b2)
{
Font n = new Font("arial",Font.BOLD,12);
l1.setText(t1.getText());
l1.setFont(n);
b2.setVisible(false);
b3.setVisible(true);
}
if(leopogi.getSource()==b3)
{
Font o = new Font("arial",Font.BOLD,16);
l1.setText(t1.getText());
l1.setFont(o);
b3.setVisible(false);
b4.setVisible(true);
}
if(leopogi.getSource()==b4)
{
Font p = new Font("arial",Font.BOLD,20);
l1.setText(t1.getText());
l1.setFont(p);
b4.setVisible(false);
b5.setVisible(true);
}
if(leopogi.getSource()==b5)
{
Font p = new Font("arial",Font.BOLD,24);
l1.setText(t1.getText());
l1.setFont(p);
b5.setVisible(false);
b6.setVisible(true);
}
if(leopogi.getSource()==b6)
{
l2.setVisible(true);
b6.setEnabled(false);
}
}
}
CLICK HERE TO VIEW APPLET SAMPLE
Jonathan Sy DIT211
///
import java.awt.event.*;
import java.awt.*;
import javax.swing.*;
import java.applet.*;
public class AppletDIT211asy extends Applet implements ActionListener
{
Button k1,k2,k3,k4,k5,k6;
Label o1,o2;
TextField p1;
public void init()
{
setVisible(true);
setSize(300,200);
setLayout(null);
setBackground(Color.blue);
k1 = new Button("Enter");
k1.setBounds(200,50,60,20);
k1.setForeground(Color.black);
k1.addActionListener(this);
add(k1);
k2 = new Button("Enter");
k2.setBounds(200,50,60,20);
k2.setForeground(Color.black);
k2.addActionListener(this);
k2.setVisible(false);
add(k2);
k3 = new Button("Enter");
k3.setBounds(200,50,60,20);
k3.setForeground(Color.black);
k3.addActionListener(this);
k3.setVisible(false);
add(k3);
k4 = new Button("Enter");
k4.setBounds(200,50,60,20);
k4.setForeground(Color.black);
k4.addActionListener(this);
k4.setVisible(false);
add(k4);
k5 = new Button("Enter");
k5.setBounds(200,50,60,20);
k5.setForeground(Color.black);
k5.addActionListener(this);
k5.setVisible(false);
add(k5);
k6 = new Button("Enter");
k6.setBounds(200,50,60,20);
k6.setForeground(Color.black);
k6.addActionListener(this);
k6.setVisible(false);
add(k6);
p1=new TextField("");
p1.setBounds(30,50,150,20);
add(p1);
o2 = new Label("you have gone over the font size limit!");
o2.setBounds(10,140,500,40);
o2.setForeground(Color.black);
Font qw=new Font("arial",Font.BOLD,15);
o2.setFont(qw);
o2.setVisible(false);
add(o2);
o1=new Label("");
o1.setBounds(120,100,120,30);
o1.setForeground(Color.black);
Font a=new Font("arial",Font.ITALIC,8);
o1.setFont(a);
add(o1);
}
public void actionPerformed(ActionEvent nathan)
{
if(nathan.getSource()==k1)
{
Font m = new Font("arial",Font.BOLD,8);
o1.setText(p1.getText());
o1.setFont(m);
k1.setVisible(false);
k2.setVisible(true);
}
if(nathan.getSource()==k2)
{
Font n = new Font("arial",Font.BOLD,12);
o1.setText(p1.getText());
o1.setFont(n);
k2.setVisible(false);
k3.setVisible(true);
}
if(nathan.getSource()==k3)
{
Font o = new Font("arial",Font.BOLD,16);
o1.setText(p1.getText());
o1.setFont(o);
k3.setVisible(false);
k4.setVisible(true);
}
if(nathan.getSource()==k4)
{
Font p = new Font("arial",Font.BOLD,20);
o1.setText(p1.getText());
o1.setFont(p);
k4.setVisible(false);
k5.setVisible(true);
}
if(nathan.getSource()==k5)
{
Font p = new Font("arial",Font.BOLD,24);
o1.setText(p1.getText());
o1.setFont(p);
k5.setVisible(false);
k6.setVisible(true);
}
if(nathan.getSource()==k6)
{
o2.setVisible(true);
k6.setEnabled(false);
}
}
}
//MELANIE MALAPITAN
//DIT211
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class AppletDIT211mam extends Applet {
int isz=8;
Font myFont;
Button button1;
TextField textField1;
public AppletDIT211mam() {
this.setLayout(null);
myFont=new Font("Times New Roman",Font.PLAIN,isz);
textField1 = new TextField();
textField1.setBounds(10, 10, 350, 40);
textField1.setText("MHELANIE MALAPITAN");
textField1.setFont(myFont);
this.add(textField1);
button1 = new Button();
button1.setBounds(10, 65, 280, 40);
button1.setLabel("CLICK TO INCREASED THE FONT");
this.add(button1);
button1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
isz+=4;
myFont=new Font("Times New Roman",Font.PLAIN,isz);
textField1.setFont(myFont);
if(isz>24) {
button1.setVisible(false);
}
}
});
}
public void init() {
}
}
Ronalyn Miral DIT211
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class AppletDIT211mam extends Applet implements ActionListener {
int i=8; Button btnRona; TextField txtRona;
public void init() {
}
public AppletDIT211mam() {
this.setLayout(null);
txtRona = new TextField();
txtRona.setBounds(15, 10, 265, 40);
txtRona.setText("Ronalyn");
txtRona.setFont(new Font("Verdana",Font.PLAIN,8));
this.add(txtRona);
btnRona = new Button();
btnRona.setBounds(15, 65, 265, 25);
btnRona.setLabel("Font Size +4");
this.add(btnRona);
btnRona.addActionListener(this);
}
public void actionPerformed(ActionEvent e) {
i+=4;
txtRona.setFont(new Font("Verdana",Font.PLAIN,i));
if(i>24) {
btnRona.setVisible(false);
}
}
}
MYRA VER ROSALES
DIT 221
Create an applet with a Button labeled "Who's the greatest?" When the user clicks the button, display your name in a large font. Class name is AppletDIT221xxx.
import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class DIT221MJR extends Applet implements ActionListener
{
Button b1;
Label lbl1;
public void init()
{
setVisible(true);
setSize(225,220);
setLayout(null);
setBackground(Color.black);
Font pogi = new Font("arial",Font.BOLD,40);
b1 = new Button("Who's the greatest?");
b1.setBounds(10,140,80,30);
b1.addActionListener(this);
b1.setForeground(Color.blue);
b1.setBackground(Color.black);
add(b1);
lbl1 = new Label("");
lbl1.setBounds(60,140,100,100);
lbl1.setForeground(Color.red);
lbl1.setFont(pogi);
add(lbl1);
}
public void actionPerformed(ActionEvent myra)
{
if(myra.getSource()==b1)
{
lbl1.setText("MYRA!!");
}
}
}
KAMLAH MYRIB CATANGUI
DIT221KMC
Create an applet with a Button labeled "Who's the greatest?" When the user clicks the button, display your name in a large font. Class name is AppletDIT221xxx.
import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class DIT221KMC extends Applet implements ActionListener
{
Button b1;
Label lbl1;
public void init()
{
setVisible(true);
setSize(225,220);
setLayout(null);
setBackground(Color.black);
Font ganda = new Font("arial",Font.BOLD,40);
b1 = new Button("Who's the greatest?");
b1.setBounds(10,140,80,30);
b1.addActionListener(this);
b1.setForeground(Color.blue);
b1.setBackground(Color.black);
add(b1);
lbl1 = new Label("");
lbl1.setBounds(60,140,100,100);
lbl1.setForeground(Color.red);
lbl1.setFont(ganda);
add(lbl1);
}
public void actionPerformed(ActionEvent kamlah)
{
if(kamlah.getSource()==b1)
{
lbl1.setText("KAMLAH!!");
}
}
}
import java.applet.*;
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
public class DIT221RAS extends Applet implements ActionListener
{
Button b1;
Label lbl1;
public void init()
{
setVisible(true);
setSize(225,220);
setLayout(null);
setBackground(Color.black);
Font rr = new Font("arial",Font.BOLD,40);
b1 = new Button("Who's the greatest?");
b1.setBounds(10,140,80,30);
b1.addActionListener(this);
b1.setForeground(Color.red);
b1.setBackground(Color.black);
add(b1);
lbl1 = new Label("");
lbl1.setBounds(60,140,100,100);
lbl1.setForeground(Color.blue);
lbl1.setFont(rr);
add(lbl1);
}
public void actionPerformed(ActionEvent rr)
{
if(myra.getSource()==b1)
{
lbl1.setText(" R-R ");
}
}
}
Post a Comment